Develop Impressive Cross Platform GUI Applications With PyQt

PyQt is a powerful Python library that allows developers to create cross-platform graphical user interfaces (GUIs) for desktop applications. It provides a comprehensive set of widgets, tools, and APIs that make it easy to build complex and user-friendly UIs for multiple platforms, including Windows, macOS, Linux, and embedded systems.
4.3 out of 5
Language | : | English |
Text-to-Speech | : | Enabled |
Enhanced typesetting | : | Enabled |
Print length | : | 1067 pages |
File size | : | 4277 KB |
Screen Reader | : | Supported |
In this comprehensive guide, we'll deep dive into the world of PyQt and explore its features, capabilities, and best practices for developing impressive cross-platform GUI applications. Whether you're a beginner or an experienced developer, this guide will provide you with all the necessary knowledge and insights to create stunning and engaging UIs for your applications.
Getting Started with PyQt
To get started with PyQt, you'll need to install it on your system. You can use pip, the Python package installer, to install PyQt using the following command:
pip install PyQt5
Once PyQt is installed, you can start creating your first GUI application. Here's a simple example of a PyQt application that displays a window with a button:
python import sys from PyQt5.QtWidgets import QApplication, QWidget, QPushButton
class Window(QWidget): def __init__(self): super().__init__()
self.initUI()
def initUI(self): self.setWindowTitle('PyQt Example') self.setGeometry(100, 100, 280, 170)
button = QPushButton('Click Me', self) button.setGeometry(100, 70, 80, 30) button.clicked.connect(self.on_click)
def on_click(self): print('Button clicked!')
if __name__ =='__main__': app = QApplication(sys.argv) window = Window() window.show() sys.exit(app.exec_())
When you run this code, it will create a simple window with a "Click Me" button. When you click the button, it will print "Button clicked!" in the console.
Exploring PyQt's Features and Capabilities
PyQt offers a wide range of features and capabilities that make it an ideal choice for developing cross-platform GUI applications. Here are some of the key features:
- Native Look and Feel: PyQt leverages the native UI elements of each platform, ensuring that your GUIs seamlessly integrate with the host operating system.
- Extensive Widget Library: PyQt provides a comprehensive library of widgets, including buttons, labels, menus, text boxes, and more, allowing you to create complex and feature-rich UIs.
- Cross-Platform Compatibility: PyQt supports multiple platforms, including Windows, macOS, Linux, and embedded systems, enabling you to reach a wider audience with your applications.
- Object-Oriented Design: PyQt follows the principles of object-oriented programming, making it easy to design, maintain, and extend your GUI applications.
- Event Handling: PyQt provides robust event handling mechanisms, allowing you to respond to user interactions, such as button clicks, mouse movements, and keyboard input.
- Database Connectivity: PyQt offers seamless integration with various database systems, enabling you to easily store and retrieve data from within your applications.
Best Practices for PyQt Development
To ensure the quality and efficiency of your PyQt applications, it's essential to follow some best practices:
- Use Qt Designer: Qt Designer is a visual design tool that allows you to create UIs visually, making it easier to prototype and design complex interfaces.
- Follow the MVC Pattern: The Model-View-Controller (MVC) pattern is a design pattern that separates the application's data model, presentation logic, and user interface, leading to increased maintainability and code organization.
- Use Qt Resources: Qt Resources allows you to bundle non-code resources, such as images, icons, and translations, into your applications, making it easier to manage and distribute assets.
- Test Your Applications: Writing automated tests for your PyQt applications ensures their stability and correctness, especially when targeting multiple platforms.
- Stay Updated with PyQt: PyQt is constantly evolving, with new features and improvements being added regularly. It's essential to stay updated with the latest PyQt versions to benefit from the latest enhancements.
Examples of PyQt Applications
PyQt has been used to develop a wide range of successful applications across various industries. Here are a few notable examples:
- VLC Media Player: VLC is a popular open-source media player that uses PyQt for its cross-platform GUI.
- Blender: Blender is a professional open-source 3D graphics creation suite that utilizes PyQt for its user interface.
- Maya: Maya is a 3D animation and modeling software that leverages PyQt for its graphical user interface.
- Inkscape: Inkscape is a free and open-source vector graphics editor that employs PyQt for its user interface.
- OpenStreetMap: OpenStreetMap is a collaborative project to create a free and open-source map of the world, with PyQt being used for its desktop GUI.
PyQt is an exceptional Python library that empowers developers to create stunning and user-friendly cross-platform GUI applications. Its comprehensive feature set, extensive widget library, and object-oriented design approach make it an ideal choice for building high-quality UIs. By following best practices, such as using Qt Designer, adhering to the MVC pattern, and staying updated with PyQt's latest advancements, you can develop robust and scalable GUI applications that meet the needs of your users across multiple platforms. With its proven track record in powering successful applications like VLC Media Player, Blender, and Maya, PyQt continues to be a trusted choice for GUI development in the Python community.
4.3 out of 5
Language | : | English |
Text-to-Speech | : | Enabled |
Enhanced typesetting | : | Enabled |
Print length | : | 1067 pages |
File size | : | 4277 KB |
Screen Reader | : | Supported |
Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Fiction
Non Fiction
Romance
Mystery
Thriller
SciFi
Fantasy
Horror
Biography
Selfhelp
Business
History
Classics
Poetry
Childrens
Young Adult
Educational
Cooking
Travel
Lifestyle
Spirituality
Health
Fitness
Technology
Science
Arts
Crafts
DIY
Gardening
Petcare
Ryan M Cleckner
Brett Hull
Steven Hugg
Michael Omi
Carol Dawson
Phil Williams
Elsevier
Jeremy J Baumberg
Nadim Saad
Kenneth Paul Rosenberg
Christopher Ketcham
Peter Wacht
Jd Tanner
Ernle Bradford
Rob Coppolillo
Kusha Karvandi
Richard Hingley
James A Whittaker
Joe Grant
Brian Noyes
Rosalyn Sheehy
Brett Cohen
Emil Frlez
Dr Nanhee Byrnes
Tom Cunliffe
Nibedit Dey
Charles Wilson
Cassandra Overby
Harold Gatty
Sara Gaviria
Alex Horne
Rachel Love Nuwer
Jacques Vallee
Hiram Bingham
Steven Shapin
Oded Galor
Brion Toss
Dusan Petkovic
Karen Wilkinson
Steve Guest
Dr Monique Thompson Dha Lpc
Renee Jain
Megan Whalen Turner
Ilchi Lee
Thomas Malory
Gerson S Sher
Pastor Ahyh
Stuart Woods
Mark Lazerus
Samuel Arbesman
Nicole Morales Lm Cpm
Cal Ripken
David Wootton
Lucia Guglielminetti
Khalid Khashoggi
Jessica Minahan
Neil S Jacobson
Miguel Crespo
Guy P Harrison
Vincent W Davis
Megan Kelley Hall
Joan Nathan
Michel Odent
Lisa Leake
Colleen Craig
Steve Oakes
John Major Jenkins
Doug Peacock
Joanna Philbin
Terence Tao
Dan Washburn
Jemar Tisby
Heyward Coleman
Cal Pater
Paula Span
Chukwuma Eleodimuo
John Connelly
Mark Seemann
Ron Lieber
John Moren
Mohammad F Anwar
Lindsey Schlessinger
Mike Eastman
Rory D Nelson
Jhenah Telyndru
Matthew Desmond
Shannon Warden
Garrett Redfield
Esther Hicks
Malcolm Hebron
Dava Sobel
David Schoem
Loyd Ellis
Eric R Dodge
Grace Friedman
Einat L K
Jonti Marks
Brett Stewart
E L Konigsburg
Eduardo Montano
Damien Cox
Kelle James
F Brent Neal
Lukas M Verburgt
Roman Gurbanov
David Hatcher Childress
Erin Mckittrick
Penny Alexander
John Wesson
Sara Elliott Price
Celina Grace
Jacqueline Houtman
Nathan Halberstadt
Christopher Clarey
John Jeffries Martin
Tim Falconer
Loan Le
Derick Lugo
Inger Mewburn
Mark J Ferrari
Inge Bell
Maggie Dallen
Salima Ikram
Maria Sharapova
Kevin Alexander
Jimmie Holland
Ann Imig
T D Wilson
Scott Hawthorn
Paula Brackston
Brian R King
Xavier Wells
Elwyn Hartley Edwards
Rahul Jandial
Warren Hansen
Jonathan H Turner
Lucas Chancel
Lewis Black
Robin Dunbar
John D Couch
Wil Fleming
Seb Falk
Robert Wright
Brogan Steele
Kerry Mcdonald
Don L Gates
Jean Nayar
Steve Wiegand
James Floyd Kelly
Jane Albert
Joanna Sayago Golub
Richard Blais
F R Lifestyle
Jason Brick
Steve Williams
Marguerite Henry
Brian Reddington
John A Fortunato
Brian Gewirtz
Charles Todd
Jacob Boehme
Nicholas Gallo
Bonnie Henderson
Peggy Kaye
Lisa Druxman
Ji Kim
Roshani Chokshi
Ursula Hackett
Clayton King
Thao Te
O S Hawkins
David Jason
Varg Freeborn
Steven Cross
Michael A Tompkins
Melinda Tankard Reist
Kent Hoffman
Jeanne Flavin
Smart Edition
1st Ed 2018 Edition Kindle Edition
Bruce A Fenderson
Sarah Lamb
Matt Schifferle
Kaylene Yoder
Catherine Shainberg
Richard A Jaffe
Kathleen Taylor
Rick Steves
Mike Adams
Elizabeth Dupart
Sandra M Nettina
Paul Farmer
Steven W Vannoy
S Connolly
Joanne M Flood
James M Jones
Cindy Margolis
Rich Osthoff
Dan Fullerton
Charlie Francis
Vicki Franz
Karl F Kuhn
John Bradshaw
Stephen Wood
Maurice Herzog
Luis Preto
Daniel Bagur
Jodi Aman
Michael Mason
Judith A Owens
Jeanne Ellis Ormrod
Matt Davids
Patrick Garbin
Keith Foskett
Worth Books
Patrick Carnes
Meg Cabot
Patti Henry
Piero Ferrucci
James Miller
Harry Vardon
Martin Sternstein
David Murray
Sam Sorbo
James D Tabor
Grace Mccready
Jeannette De Wyze
Larry A Yff
Kate Mcmillan
Grete Waitz
Kathleen Cushman
Curtis Wilkie
George Case
Keith Ammann
Daniel Bergner
Cheryl Diamond
Douglas Wood
Nick Heil
Natalia Ilyin
Jameswesley Rawles
Joanna Faber
Gene Kritsky
John Monaghan
Soong Chan Rah
Richard Bass
Colleen Doyle Bryant
Dave Cutcher
Keith Ryan Cartwright
Samantha Michaels
John Fraser Hart
Ted Franklin Belue
Elizabeth Davis
Pat Manocchia
Bret Stetka
Gerald L Schroeder
Derek Blasberg
Scott Reed
Nachole Johnson
David M Ewalt
Scott Zimmerman
Karen Ward Mahar
Molly Caldwell Crosby
Steven Trustrum
Eric Michael
Mary Morrison
Michael Shaw
Tyler Hamilton
Grace Mariana Rector
Eze Ugbor
Zigzag English
Frederick L Coolidge
Michael Sullivan Iii
Kevin Marx
Joyce Bas
Genie Reads
Douglas R Hofstadter
George Olsen
Christine Brennan
Jp Kriya
Marc Bona
Susan F Paterno
Gerald R Allen
Jennifer Comeaux
Wilborn Hampton
Karen E Mcconnell
Nora Roberts
D Levesque
Sarah Kleck
Edith Hall
Geoffrey Simpson
Nate Allen
Patty Wipfler
Gjoko Muratovski
Peter Allison
Porter Shimer
William Albert Robinson
David Cannon
Guido W Imbens
Tobe Melora Correal
Debra Fine
Gabriel F Federico
Htebooks
Nicholas S Howe
Stephanie Perkins
St Teresa Of Avila
Megan Davidson
Dinesh Kumar Goyal
Dr Alison Dibarto Goggin
Howtodressage
Jackie Freeman
Mick Conefrey
Mary Strand
J F James
James Kaiser
Mark Johnston
Peter Zuckerman
Bruce Lee
Jeannie Burlowski
J C Cervantes
Lee Alan Dugatkin
Tadashi Yoshimura
Lana Peek
Ethan Bezos
Jason Sandy
Kyle Rohrig
Calvin Trillin
David Flanagan
Pittacus Lore
Steven Verrier
Paul Simpson
Courtney Macavinta
Lori Lyons
Sandy Jones
Jay Asher
Rachel Mcgrath
Brian Everitt
Dr Faith G Harper
Gustav Meyrink
Bruce Macdonald
Lisa Scottoline
G E R Lloyd
Natalia Rojas
Genevieve Bardwell
Martin Odersky
Laura A Jana
Helen Zee
P A Johnson
Martin A Lee
Jp Lepeley
Christy Teglo
Collins O Onwe
Bryan Berard
Sunil Tanna
Breanna Hayse
Mahmood Mamdani
Adrienne Onofri
Arthur Scott Bailey
Michael Sean Comerford
Scott Jurek
Joseph Burbridge
Adam Night
Chuanwei Li
Brian Herne
Jim White
Claudia M Gold
Laurie A Watkins
Megan Smolenyak
Brittany Cavallaro
John Mcenroe
Nicholas Bjorn
Melanie Challenger
Julie Cangialosi
Carlo Zen
Matt Parker
Colette Harris
Sonja Schwartzbach
Lucinda Scala Quinn
Nancy Boyd Franklin
Deborah Shouse
Lee Mcintyre
Luis Angel Echeverria
Brienne Murk
Mitchel P Roth
Diana J Mason
Marc J Reilly
Avery Faigenbaum
Lucy Hopping
Manfred Theisen
Phyllis Books
Stephanie Sarkis
Mark Powell
Kate S Martin
Fritjof Capra
Timothy A Sisemore
David Hoffman
David Levithan
Ksenia K
Emilee Day
David Sinclair
Sean Michael Wilson
Rob Collins
Daniel Dell Uomo
Peggy Tharpe
Jaime Flowers
Steve Bromley
Ivan Savov
Jennifer Block
Emily Lauren Dick
David Hackett Fischer
William Shakespeare
David Mcclung
T J Tomasi
Victor A Bloomfield
Cpt Exam Prep Team
Matt Wastradowski
Rick J Scavetta
Caitlin Flanagan
Thomas A Jacobs
Rob Vollman
Mitt Romney
Janae M Robinson
Chase Hassen
Pedro Sarmiento De Gamboa
Kindle Edition
Jackson T Markbrown
Henry Worsley
Denise Long
Matt Doeden
Carol Newell
Jeremy Bradstreet
Roach Mary
Cecilia Twinch
Brooks Blevins
Florence Weiser
Kazumi Tabata
Richard Post
M A Hayat
Martin Wells
William Trubridge
Ned Mcintosh
Kicki Hansard
E G Richards
Della Ata Khoury
Harry Bauld
Brian Kilmeade
Danny Staple
Heather Rose
Kara Forney
Nehemia Gordon
Mark Howard
Caitlyn Dare
Rich Cohen
James O Prochaska
Krishna Godhania
Jules Wake
Masaaki Kijima
Deepak Chopra
Patricia Wooster
Dan Jones
Paul Carus
J R Mathews
Jonathan Grix
Matt Morton
Michael Barkun
Janice L Raymond
Steven Emanuel
Kira Breed Wrisley
William Souder
Dr Lena Edwards
Sriman Sharma
Frederick Grinnell
Robert Ullman
Steve Schwartz
Dave Ramsey
Scott Haines
Shayla Black
Hal R Varian
Eric C Lindstrom
Toni Weschler
Juliet Miller
Bernard Darwin
Dawna Markova
Richard Kasper
Mary Heffernan
James Zug
Milne Cc Pocock
Neil Postman
David Barrett
Clyde Soles
Melissa Trevathan
Brian W Kernighan
Tim Dunn
Samuel Greenberg
Alan D Moore
Jay H Lefkowitch
Michael Masters
J R Harris
J Wayne Fears
Ian Tuhovsky
Ronald M Rapee
Cecelia Ahern
Carol Walters
D Enette Larson Meyer
Joseph Ewing
Tom M Apostol
Michael Ruhlman
Judy H Wright
John M Taylor
Santari Green
James Dean
Mike Weatherstone
Joel Ingersoll
Lenore Skenazy
Murray Shukyn
Kalynn Bayron
Leslie Valiant
Colleen Houck
Marco Polo
Daniel Vaughan
Leigh Pearson
Lily Raff Mccaulou
Iwan Rhys Morus
Estelle Dautry
Michael O Emerson
Elliot Davis
Mariana Monteiro
Joyce Harper
Phillip Stephen Schulz
Paul Weamer
Don Brown
David Barrie
D S Malik
Chuck Callaway
Jez Cajiao
Mark William
Bobbi Conner
Sujit Sivasundaram
Carol Kaesuk Yoon
Trish Kuffner
John Cooper
John Mclachlan
Claudia Gray
Sue Wieger
Kathleen Bartholomew
Diane Ravitch
Linda Carter
Stephen Hawking
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!
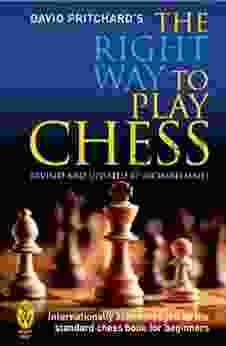

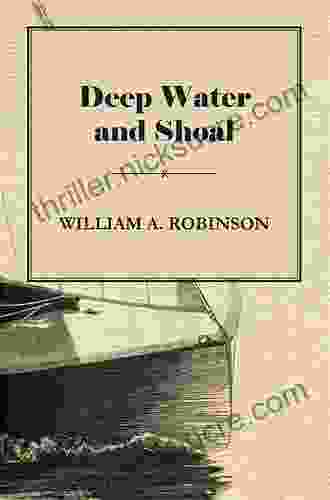

- Glenn HayesFollow ·11k
- Charlie ScottFollow ·15.3k
- Galen PowellFollow ·13.2k
- Harold BlairFollow ·11.1k
- Tennessee WilliamsFollow ·5.9k
- Clarence MitchellFollow ·3.3k
- Frank ButlerFollow ·17.7k
- Mario Vargas LlosaFollow ·7.1k
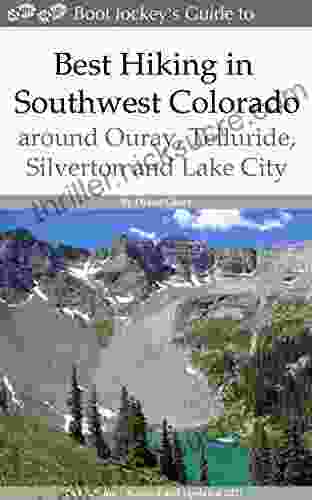

2nd Edition Revised And Expanded 2024: A Comprehensive...
The 2nd Edition Revised...
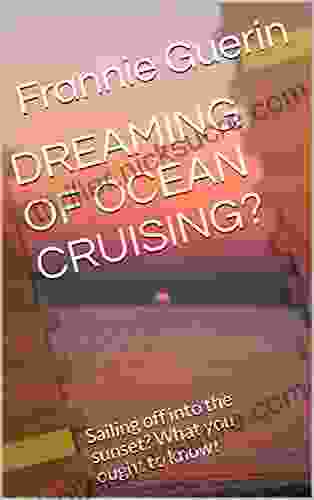

Dreaming of Ocean Cruising: A Voyage into Tranquility and...
For those seeking a respite from the mundane...
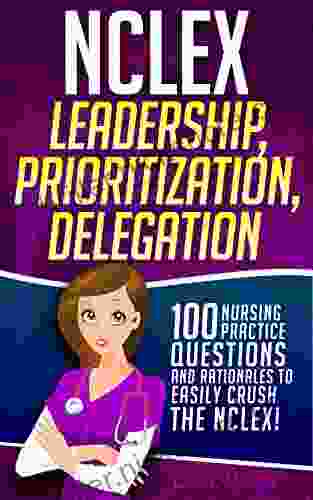

100 Nursing Practice Questions with Rationales to...
The NCLEX exam is a challenging but...
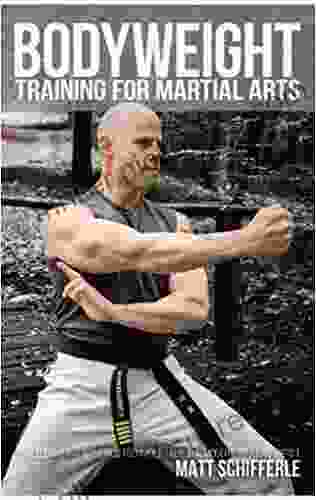

Mastering Bodyweight Training for Martial Arts: A...
For martial...
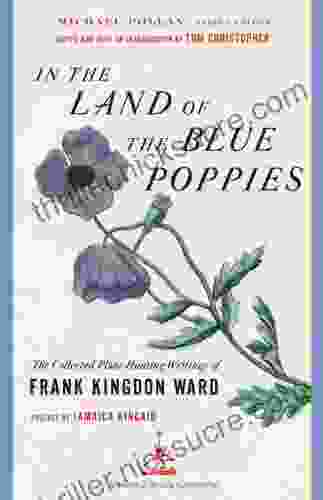

In The Land Of The Blue Poppies: A Literary Journey to...
Prologue: A Tapestry of...
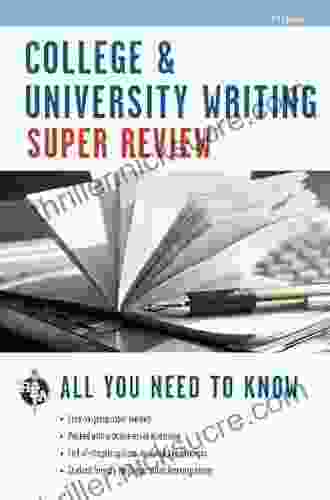

College University Writing Super Review Flash Card Books:...
College University...
4.3 out of 5
Language | : | English |
Text-to-Speech | : | Enabled |
Enhanced typesetting | : | Enabled |
Print length | : | 1067 pages |
File size | : | 4277 KB |
Screen Reader | : | Supported |